In Part 1 we set up Firebase and in Part 2 we added user registration and login. In this part we are going to see how to use a database.
Setting Up
Head over to your Firebase console and click on Cloud Firestore. Then click “Create Database”. Select “test mode” and “Enable”.
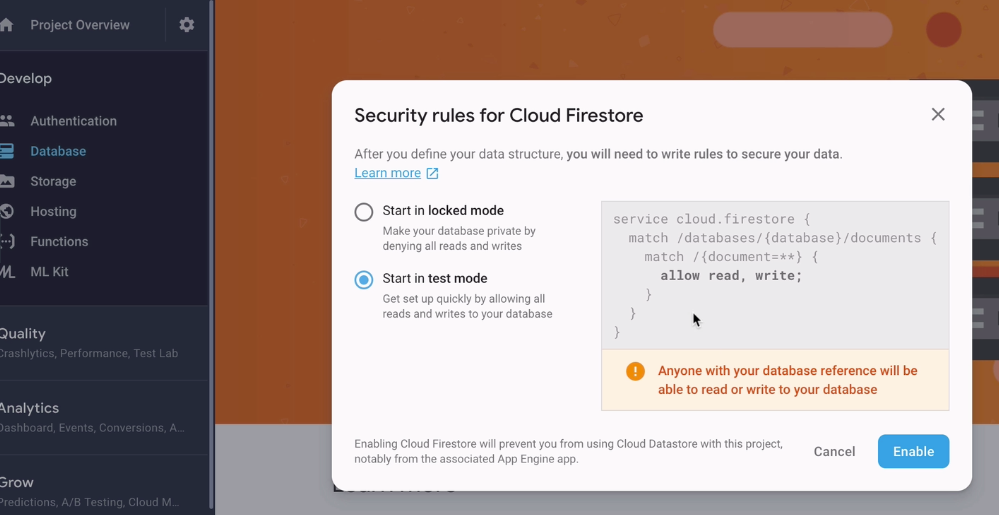
Next we need to create a collection.
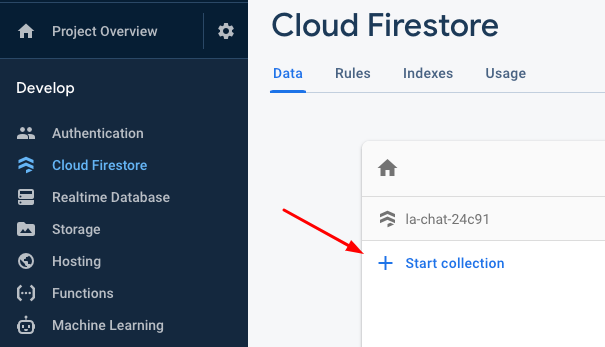
Next we need to give a Collection ID
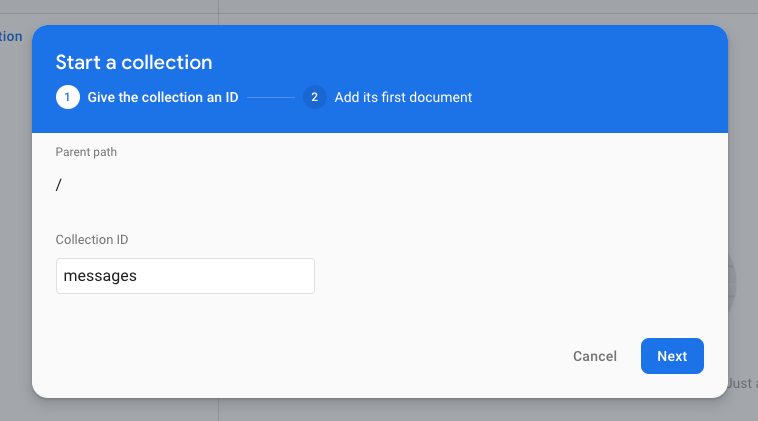
We can then add some fields (I had to click Auto-ID, otherwise I got the red box)
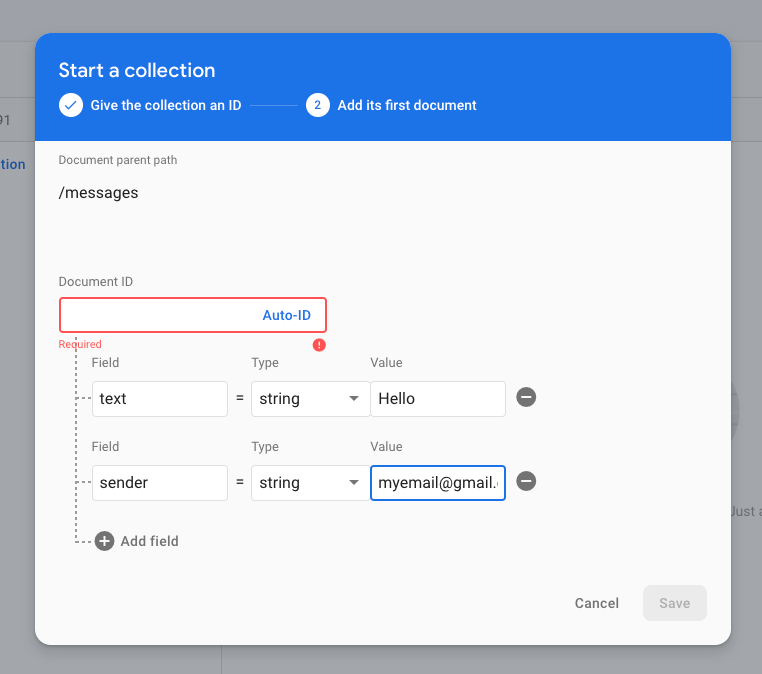
And once we save we should have our collection.
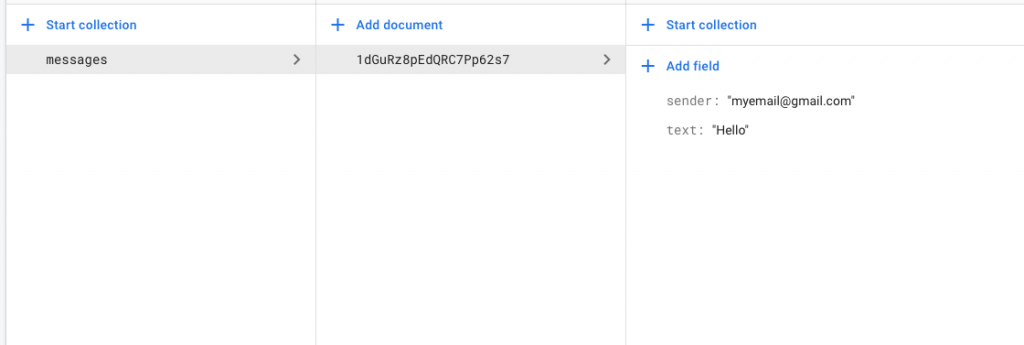
Saving Data
Next, we are going to access this collection from our app. First we import it
import 'package:cloud_firestore/cloud_firestore.dart';
and grab the instance of the singleton:
final _firestore = FirebaseFirestore.<em>instance</em>;
To send a value to the collection all we have to do is the following:
_firestore.collection('messages').add({
'text': messageText,
'sender': loggedInUser.email,
});
We just have to make sure to get the collection name and the field names right. Once you send a message using this code, you should see it show up in your collection:

Getting Data
As you might have guessed, getting data is pretty much as straightforward as saving it:
final messages = await _firestore.collection('messages').get();
for (var message in messages.docs) {
print(message.data());
}
Here data will be a bunch of key value pairs that looks like:
flutter: {text: "adsf", sender: myemail@gmail.com}
The above method is a simple get request. What we might want is to subscribe to a stream that pushes data to us as it’s added. In that case we can do this:
void stream() async {
await for (var snapshot in
_firestore.collection('messages').snapshots()) {
for (var message in snapshot.docs) {
print(message.data());
}
}
}
Now any time data is added this function will run again. This auto subscribes to Firebase and the loop is run every time data is added. Now we can do something like use a StreamBuilder to build our views easily.
StreamBuilder<QuerySnapshot>(
stream: _firestore.collection('messages').snapshots(),
builder: (context, snapshot) {
if (snapshot.hasData == true) {
List<Text> messageWidgets = [];
final messages = snapshot.data.docs;
for (var message in messages) {
messageWidgets.add(Text(message.data()['text']));
}
return messageWidgets;
} else {
return Center(
child: CircularProgressIndicator(),
);
}
}
)